Plotting I-V Curves using Python
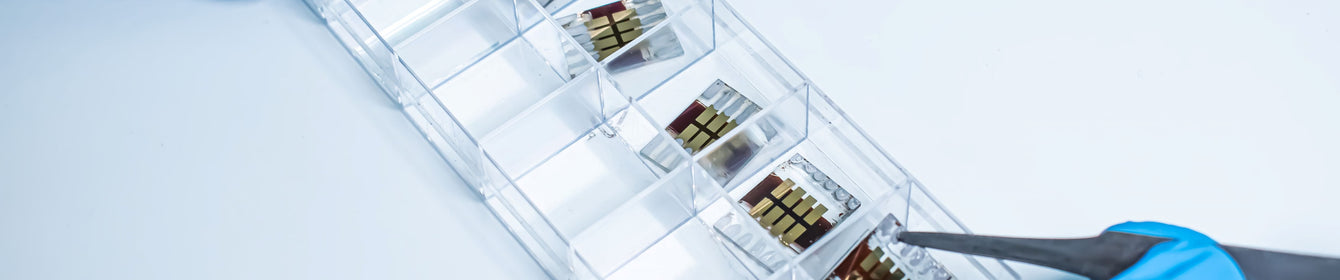
Ossila Solar Cell I-V software (compatible with the Ossila Solar Cell IV Test System) saves I-V data (or J-V data) in the following form.

This data can be easily plotted using data visualisation software such as Excel, Origin or SciDAVis. You can also use the following Python code to plot this data using Panda DataFrames. Just copy and paste the code below into your Python virtual environment and start plotting.
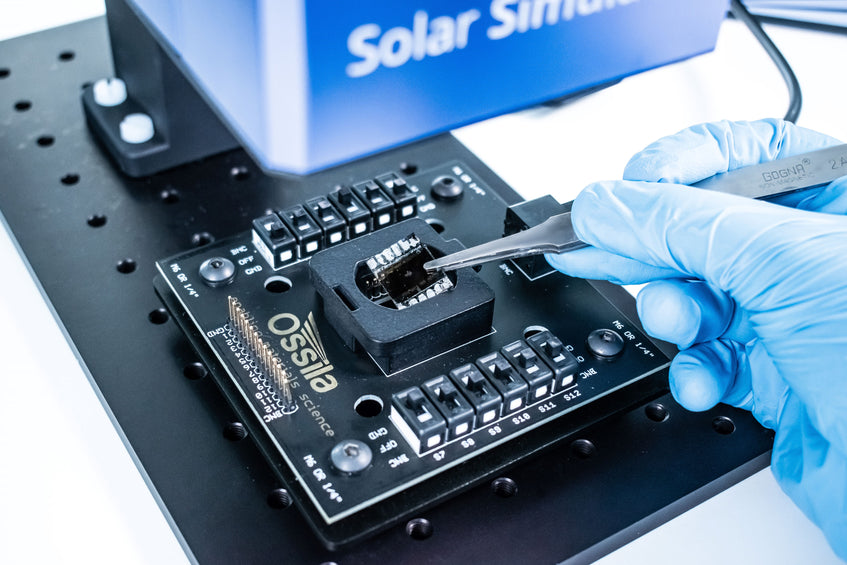
Solar Cell Testing Kit
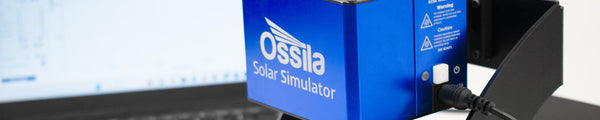
Python Code for I-V Curves
This section of code can be used to read an Ossila I-V Data .csv into a Panda DataFrame and prepare the data for plotting.
#%%
# Import the following packages
# These packages are included with Python
import csv
from pathlib import Path
from tkinter import filedialog
# These packages need to be installed using pip
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# Read in .csv file as a DataFrame
file_path = filedialog.askopenfile()
data = pd.read_csv(file_path, skiprows=1, engine='python')
#%%
# This cell will define the headers for your DataFrame
# Find the number of pixels on your device
number_of_pixels = int(data.shape[1] / 4)
# Create a list of column names: the Forward + Reverse sweep for each pixel
labels = []
for i in range(1, number_of_pixels + 1):
labels.append(f'Pixel {i} Forward V')
labels.append(f'Pixel {i} Forward J')
labels.append(f'Pixel {i} Reverse V')
labels.append(f'Pixel {i} Reverse J')
# relabel the column heads
data = data.set_axis(labels, axis='columns')
#%%
# Convert J from A/cm2 to mA/cm2
for i in range(1, number_of_pixels + 1):
data[f'Pixel {i} Forward J'] = data[f'Pixel {i} Forward J'] * 1000
data[f'Pixel {i} Reverse J'] = data[f'Pixel {i} Reverse J'] * 1000
#%%
# Define colours if needed in this cell
# One needed for each pixel if you are using
colours = ['#384A9C', '#418FDE', '#AC1E2C', '#54565A', '#CCD4EC', '#0C9748', '#ffc125', '#000000']
You can plot the I-V sweep of an individual pixel using the code below.
#%%
# This cell will plot the forwards and reverse sweep for a specific defined pixel
# Define the pixel you want to plot
which_pixel = input('Please enter the pixel you want to plot.\n')
# Plot the J-V curve
# If not defining colours, remove "colour=colours[0]"
plt.plot(
data[f'Pixel {which_pixel} Forward V'],
data[f'Pixel f{which_pixel} Forward J'],
color=colours[0],
linewidth=1,
label='Forward',
)
plt.plot(
data[f'Pixel {which_pixel} Reverse V'],
data[f'Pixel f{which_pixel} Reverse J'],
'--',
color=colours[0],
linewidth=1,
label='Reverse',
)
# You can change the code below to change the appearance and scale of your graph
# Possible changes can be found in the matplotlib library
plt.axvline(x=0, color='black', linewidth=0.5)
plt.axhline(y=0, color='black', linewidth=0.5)
plt.xlabel('Voltage (V)')
plt.ylabel('Current Density (mA/$cm^{2}$)')
plt.legend(loc=0)
plt.show()
This section of code will produce a graph like the one below.
Alternatively, you can use this code to plot the J-V sweep for every pixel on a device.
#%%
# This code will plot the J-V sweep for all pixels on your device
# Plotting each pixel
# If not defining colours, remove "color=colours[i - 1]"
for i in range(1, number_of_pixels + 1):
plt.plot(
data[f'Pixel {i} Forward V'],
data[f'Pixel {i} Forward J'],
color=colours[i - 1],
linewidth=1,
label=f'Pixel {i} Forward',
)
plt.plot(
data[f'Pixel {i} Reverse V'],
data[f'Pixel {i} Reverse J'],
'--',
color=colours[i - 1],
linewidth=1,
label=f'Pixel {i} Forward',
)
# You can change the code below to change the appearance and scale of your graph
# Possible changes can be found in the matplotlib library
plt.axvline(x=0, color='black', linewidth=0.5)
plt.axhline(y=0, color='black', linewidth=0.5)
plt.xlim(-0.1, 1.2)
plt.ylim(-25, 35)
plt.xlabel('Voltage (V)')
plt.ylabel('Current Density (mA/$cm^{2}$)')
plt.legend(loc=0)
plt.legend(ncol=2)
plt.show()
Solar Cell Testing Kit
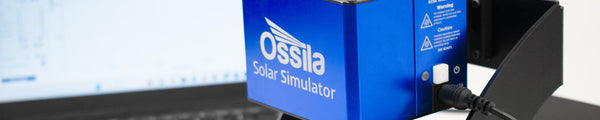
Module List
Details and licensing information on of the modules used in this code is provided below.
Matplotlib
Matplotlib is a library that is used in Python to easily create a display various forms of charts and graphs. Plotting is as simple as calling plot(x_values, y_values)
in most cases. More can be seen in our tutorials on matplotlib.
Pandas
Pandas is a python package that simplifies working with "labelled" or "relational" data, making real-world data analysis faster and easier. Processing files using DataFrames can make data handling, plotting, and analyzing uncomplicated and simple.